Introduction: City With Glowing Light
For this contest, I decided to make a city with a glowing light in the background. I was using Arduino Uno.
Supplies
Supplies:
1 – Box (include the scenery inside)
1 – Arduino Uno Board
6 – Jumper Cable
2 – 220 Ω Resistors
2 – 10 kΩ Resistors
1 – Pushbutton
1 – Red LED
1 – Blue LED
1 – Breadboard
1 – USB Arduino Connector
Step 1: Making City's Skyline Using Python Matplotlib:
I made the box and the city. For graphing the city's towering building, I used a Python module called Matplotlib. Here I embed the code below for Python Matplotlib:
----------------------------------------------------------------------------------
from matplotlib import pyplot as plt
# Create histogram (representing city's skyline):
my_dpi = 200
fig = plt.figure(figsize=[800*11/8.5/my_dpi, 800/my_dpi], dpi=my_dpi)
num_bins = [0,9,10,19,20,29,30,39,40,47.5,50,59,60,66,67,76]
n, bins, rects = plt.hist([], num_bins, ec='k')
heights = [10, 3, 7, 3, 12, 3, 30, 3, 13, 3, 13, 3, 25, 3, 20, 3, 15, 3, 18]
for r in range(len(rects)):
rects[r].set_height(heights[r])
rects[r].set_color([0.86, 0.86, 0.76, 1])
plt.ylim(1,31)
plt.axis('off')
fig.savefig('City Skyline.jpg', dpi=my_dpi)
fig.show()
I will explain generally the code above.
First, I imported the Python library Matplotlib. Then, I created histogram that is representing city's skyline. I changed the rectangles' widths (bins) and heights as I desired.
Step 2: Building the Circuit:
Here is the schematic of the electronic circuit.
I built the circuit using Arduino Uno. I used 2 LEDs, which are
red and blue, 4 resistors, which are 2 of 220 Ω Resistors and 2 of 10 kΩ Resistors. I plugged all of electronic components in the breadboard and connected all them to the Arduino Pins. By using USB, I plugged the Arduino Board into my computer. Arduino Uno circuit board is also programmable. Here I embed the code below for Arduino programming:
----------------------------------------------------------------------------------
int buttonPin = 12; // the number of the push button pin
int led1Pin = 9; // the number of the LED pin
int led2Pin = 8; // the number of the LED pin
boolean isLighting = false; // define a variable to save the state of LED
void setup() {
pinMode(buttonPin, INPUT); // set push button pin into input mode
pinMode(led1Pin, OUTPUT); // set LED pin into output mode
pinMode(led2Pin, OUTPUT); // set LED pin into output mode
}
void loop() {
if (digitalRead(buttonPin) == LOW) { // if the button is pressed
delay(10); // delay for a certain time to skip the bounce
if (digitalRead(buttonPin) == LOW) { // confirm again if the button is pressed
reverseLED(); // reverse LED
while (digitalRead(buttonPin) == LOW); // wait for releasing
delay(10); // delay for a certain time to skip bounce when the button is released
}
}
}
void reverseLED() {
if (isLighting) { // if LED is lighting,
digitalWrite(led1Pin, HIGH); // switch on LED
digitalWrite(led2Pin, LOW); // switch off LED
isLighting = false; // store the state of LED
}
else { // if LED is off,
digitalWrite(led1Pin, LOW); // switch off LED
digitalWrite(led2Pin, HIGH); // switch on LED
isLighting = true; // store the state of LED
}
}
----------------------------------------------------------------------------------
Step 3: Implementation:
I tried my electric circuits. When my Arduino connects to my computer, this meant that there is a flow of electricity from my computer to my Arduino and electronic components. First, both of the lights were off. When I pushed the button, the red LED light bulb turned on. When I pushed the button again, the red LED light bulb turned on, and the blue LED light bulb went off. Pushing the button again, the blue LED light bulb switched on, the red LED light bulb turned off, and so on.
I hope you will like this project. Please kindly vote for me.
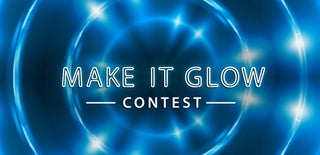
Participated in the
Make it Glow Contest